Introduction
Servlet filter provides a filter layer between the client and the servlet. When the client invokes the request, at that time servlet filter will perform the pre-process steps to filter the request before completing the request, also it will perform the post-processing steps before sending it to the client.
Here are some common filter usage cases.
- Logging
- Auditing
- Transaction management
- Security
1) Create a module project.
- Go to Liferay workspace project → modules → new.
- Select other → Liferay → Liferay Module Project and Click on “Next”.
- Enter the project name.
- Select “Project Template Name” as “war-hook” and click on “Next”.
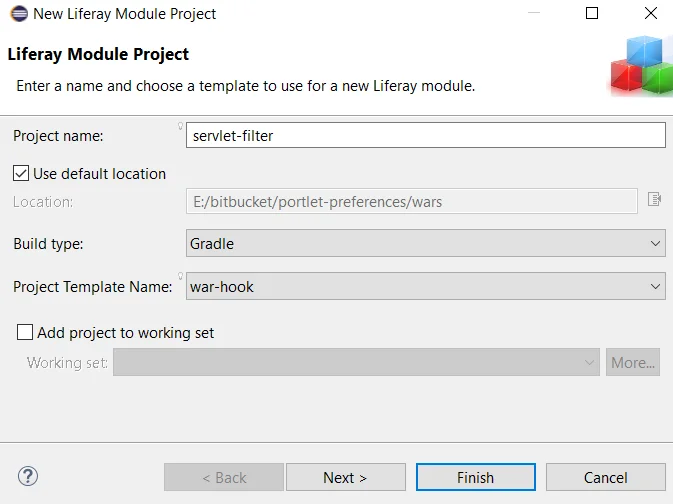
- Enter a Package name and click on the “Finish”. The necessary file structure will be created as below.

2) Create a servlet filter class and implement with the Filter class.
// ServletFilter.java
package com.ignek.portal.web.hook;
public class ServletFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
System.out.println("Called ServletFilter.init(" + filterConfig + ") where hello="
+ filterConfig.getInitParameter("hello"));
}
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse,
FilterChain filterChain)throws IOException, ServletException {
String uri = (String) servletRequest.getAttribute(WebKeys.INVOKER_FILTER_URI);
System.out.println("Called ServletFilter.doFilter(" + servletRequest + ", "
+ servletResponse + ", "+ filterChain + ") for URI " + uri);
filterChain.doFilter(servletRequest, servletResponse);
}
@Override
public void destroy() {
}
}
Filter interface provides the following life cycle methods.
- init(): It is used to initialize the filter.
- doFilter(): It is used to perform filtering tasks.
- destroy(): Clean up the filter’s unneeded resources.
3) Add below mapping in Liferay-hook.xml.
// liferay-hook.xml
portal.properties
ServletFilter
com.ignek.portal.web.hook.ServletFilter
hello
world
ServletFilter
/group/*
/user/*
/web/*
*.jsp
REQUEST
FORWARD
4) Now, you can deploy it in the Liferay server and observe the logs in the terminal(cmd).
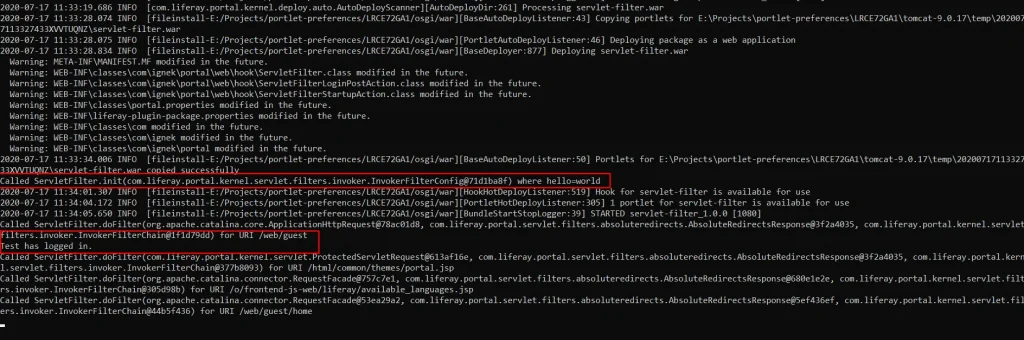